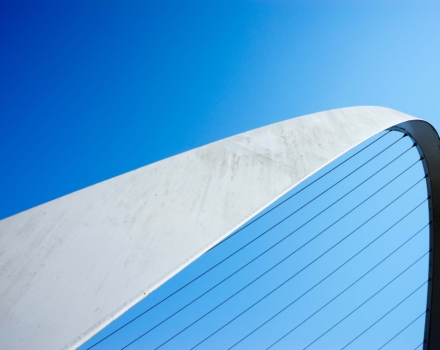
Architecture
The TOGAF® Standard, 10th Edition The Open Group has released a new Standard, version 10:…
Read more
There are different ways to handle data tables in Cucumber scenarios. Which method of data table you use depends on your project and complexity of your test. In this article, I will show some methods with their examples. I will also discuss the advantages and disadvantages of each method. In the end, it is up to you to decide which method best suits your needs.
You can add data to your Gherkin as a list. This method is best used when you have a small data set of the same data type and the order of the data is unlikely to change or not important (for example: a list of numbers that you want to loop through).
For example:
In your step file, this data can be read as:
This is probably the simplest method to implement data tables. In this case, we have 3 string values. Instead of strings, you can switch the data type of the list to any data type that you want. However, the disadvantage of this method is that you can only use a single data type, so only strings or only integers, etc. You cannot mix the different data types into the same list. Furthermore, you are dependent on the order of your data in your list. If you want to add an item to a position other than the end of the list, you will need to update your indexes of the rest if each data point has its own function.
Summary:
+ Simple to implement
– Limited to single data type in list
– You have to keep track of the order of your data in the list
Instead of a list, we can also use a map. This solves the problem of keeping track of the order of the data in your list. This method is best used when you have a small/medium data set of the same data type and each data value has its own function.
In Gherkin this would look like:
In your step file, this data can be read as:
Because we are now using a map, the order of the data is no longer an issue. We can access the data by simply calling the key which is the first column of the data. However, we can still only have the same data type in the entire map. In this case <String, String>. Keep in mind that keys in the map need to be unique.
Summary:
+ Fairly simple to implement
+ The order of your data is not an issue
– Limited to single data type in map
You can also create a custom object for your data table. A great advantage of using this method is that you can handle different data types in your data table. For example: if we want to create data for a product in a store, we can create an object called Product.
In Gherkin we can give values to each field of the Product object:
It is important that the names in the top row are an exact match with your field names of your custom object. Cucumber is only forgiving in capitalization of the first letter. Also note that the topmost row now has the “keys” and the second row contains the data where in method 2 the first column was the key and the second column the value.
In your step file, this data can be read as follows:
In order to access the fields, we need to add getters to the Product class. So the final Java file looks like:
With the getters in place, we can now access the fields in our steps. For example:
As you can see, we can now use multiple data types in our data input (strings, integers and doubles in our case). Another advantage is that you can work with multiple data objects like this:
And access them all in the steps file with for example a loop:
Summary:
+ Multiple data types possible
+ The order of your data is not an issue
+ Multiple data objects in a single step
– Bit more complex to implement
This is a more complex usage of data tables, where I no longer wanted to create new test data every time I ran my test. It is actually an extension of method 3. This method is highly recommended when working in a test environment where your test data has to be unique each time you run your test (for example when filling in forms) or when working with large data sets. It allows you to control the values that you want and randomize other values.
Like we did in method 3, we need to create an object. But we now add a Constructor which generates our fake data. I use the Java Faker package to generate the fake data. For example:
In Gherkin we give values only to the fields that we want to control the values of:
And then in our steps file, we generate the rest of the data:
As you can see I am calling the Java method generateRemainingData in ContactFormData. You can add this method to the ContactFormData class with the following code:
This Java method calls the generic Java method in my DataHelper class (which we have not yet created). In calling this method, it passes on the initialData that I filled in in my Gherkin, and it passes on a new data object using the constructor that generates the fake data. So all we need now is the generic generateRemainingData method somewhere (In my case, it is located in a class called DataHelper. You can place this method anywhere you want). This generic method only needs to be defined once for your entire project, since it works with generic class types.
This method for data tables in Cucumber is also very handy when you have large data sets. Since you only fill in some fields in your Gherkin and generate data for the rest of the fields, your feature file is not cluttered with unnecessary information.
Summary:
+ Possibility to generate fake data
+ Multiple data types possible
+ The order of your data is not an issue
+ Multiple data objects in a single step
– Complexity of code
Expert: Mazin Inaad, trainer Capgemini Academy
You can find all our Java courses on this page. Please don’t hesitate to reach out to us if you have any questions regarding Java, or any of our courses.